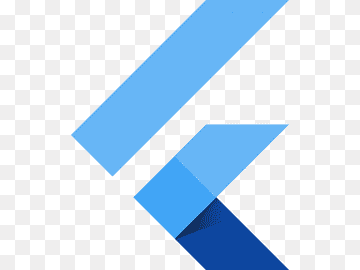
Flutter
Make the most of this cutting-edge technology by developing apps quickly! Our Flutter solutions have amazing features that can be used to create sleek, high-performance apps that can scale seamlessly across platforms.
Flutter is increasingly becoming a top choice for cross-platform app development. One key challenge when building Flutter apps is state management – tracking changes and updating the UI. In this guide, we’ll compare popular state management solutions like setState, BLoC, RxDart, and more.
In reactive frameworks like Flutter, the UI is rendered based on variable state.
Without a proper state management system, it’s easy to end up with disjointed code and a poor user experience. Common problems include:
That’s why choosing an optimal state management approach is critical for Flutter. It will impact your code architecture, app performance, testing, and Iphone app developers Kerala productivity.
The basic way to manage state in Flutter is with setState(). This method marks the widget state as dirty, triggering a rebuild.
Here is an example with a counter variable:
int counter = 0;
void increment() {
setState(() {
counter++;
});
}
setState is simple and works for basic apps. But it leads to code duplication for anything complex. Our logic ends up split between widgets and can be difficult to track.
For Flutter state management, BLoC (Business Logic Component) pattern is a preferred option.
It separates presentation from logic by extracting it into BLoCs. These handle the app’s state and logic flows:
Widgets dispatch events to the BLoCs, which update the app state. The new state flows back to the UI which rebuilds accordingly.
Key advantages of BLoC:
BLoC libraries like flutter_bloc make implementation straightforward. Overall, BLoC scales well and helps manage complexity in larger Flutter apps that falls in the Best mobile application development Kerala, category.
RxDart brings reactive streams to Flutter by combining rxdart and streams.
State is managed as an observable data stream using Rx primitives like Subjects, combineLatest, etc.
Here is an example with a CounterBloc:
class CounterBloc {
final _counter = BehaviorSubject<int>();
Stream<int> get counter => _counter.stream;
void increment() => counter.add(counter.value + 1);
}
The UI subscribes to the counter stream. When increment is called, it updates the stream which rebuilds widgets.
RxDart offers powerful reactive capabilities for state management. However, it has a steep learning curve which makes BLoC more accessible for many teams
Here are some other popular state management libraries for Flutter:
Provider – Built-in simple state management using ChangeNotifier and Consumer/Selector widgets. Good for basic needs.
Redux – Port of the popular Redux architecture from React to Flutter. Uses central store approached.
MobX – Implements observable states with actions, reactions and computed values. ReactJS-based.
GetIt – Simple service locator that allows sharing state between widgets.
Each library has tradeoffs to consider for app architecture, performance, and testing needs.
Here are some best practices when selecting a state management solution:
App complexity – Simple apps may need only setState or Provider, while larger apps benefit from BLoC or Redux.
Team experience – Leverage existing knowledge your team has with a given architecture.
Code reuse – Some patterns like BLoC and Redux promote reusability better.
Testing – Certain methods like BLoC and Redux are more testable than others.
Performance optimization – Solutions like RxDart offer fine-grained control over rebuilding.
Well-organized state management is critical for Flutter apps. Approaches like setState, BLoC, and RxDart each have their own strengths.
Consider your app requirements, team skills, and architecture goals when selecting a state management solution. This will set your Flutter project up for success.